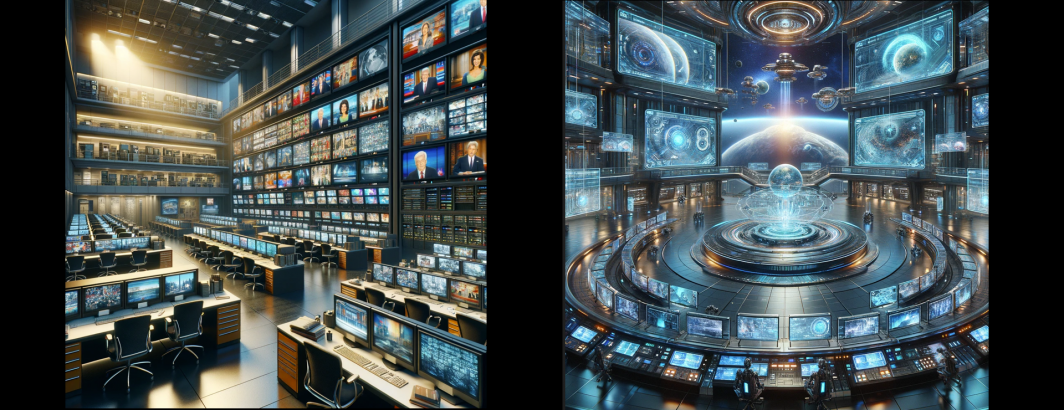
One of the more fascinating aspects of our experiments applying LLMs as part of our technical solutions and coding workflows is the degree to which they tend to favor vastly more complicated solutions than what might make the most sense in any given scenario. For example, recently we had the following challenge:
I have to download millions of large MP4 video files using a cluster of cloud VMs. There is a fixed collection of six million historical videos, plus a few thousand new videos being added each day. Each VM runs 24 downloading threads and cannot run more than that. There is a central queue into which the list of videos to download is steadily being fed and all of the VM downloading threads poll this queue each time they complete a download to get the next video to download. My problem is that the queue can quickly become overloaded if I just submit a fixed batch size of videos every X minutes. Describe to me a basic algorithm that could submit videos to the queue such that all of the videos are downloaded as quickly as possible, but don't overwhelm the queue.
While ChatGPT provides a range of reasonable responses, its answers trend towards more complex solutions and are presented with accompanying mathematical formulas and assumptions that fail to respond to the core of the underlying use case: downloading large files via a cluster with a fixed number of threads per node, which implies that each download will take on the order of tens of seconds to minutes or tens of minutes, rather than microseconds, meaning queue draining will occur at a relatively manageable rate. This suggests that the simplest and most reasonable solution to the challenge posed above is to simply maintain a fixed queue size, automatically adding records as needed to maintain a specific size. ChatGPT largely skips over this most trivial of solutions and even pushes back on it when presented with it as the expected answer, arguing that in order to "maximize the utilization of the VMs and ensure the fastest possible download times, a more sophisticated algorithm that dynamically adjusts to real-time conditions might be more appropriate." Bizarrely, it connects queue size to download time, reinforcing that contemporary LLMs are merely statistical autocompletion engines that cannot actually reason about the world.
The first output from ChatGPT featured multiple mathematical formulas:
To efficiently manage the submission of videos to the queue without overwhelming it, you can implement a dynamic rate-limiting algorithm that takes into account the current load on the queue and the processing capacity of your VMs. Here's a basic algorithm outline:
### Algorithm Outline: Dynamic Rate-Limited Submission
1. **Initialize Variables:**
– Set an initial batch size, `B0`, for the number of videos to submit to the queue at the start.
– Define a maximum batch size, `Bmax`, and a minimum batch size, `Bmin`.
– Set an initial submission interval, `T0`, which is the time delay between consecutive batch submissions.
– Define a maximum interval, `Tmax`, and a minimum interval, `Tmin`.
2. **Monitor Queue Status:**
– Continuously monitor the queue's load, `Q`, which is the number of videos currently in the queue.
– Monitor the average processing speed of the VMs, `S`, which could be measured as the average number of videos downloaded per second across all VMs.
3. **Calculate the Ideal Submission Rate:**
– Calculate the ideal submission rate based on the processing speed:
\[
R_{\text{ideal}} = S \times N_{\text{VMs}} \times \text{Threads per VM}
\]
– The ideal submission rate (`Rideal`) should aim to keep the queue at a level where it has enough work for the VMs but is not overloaded.
4. **Dynamic Adjustment of Batch Size and Interval:**
– Adjust the batch size based on the current queue size and processing speed:
\[
B_{\text{next}} = \min\left(\max\left(\frac{R_{\text{ideal}} \times T0}{Q + 1}, B_{\min}\right), B_{\max}\right)
\]
– If the queue size is low (indicating the VMs are quickly processing the videos), increase the batch size slightly and reduce the interval:
\[
T_{\text{next}} = \max\left(\frac{B_{\text{next}}}{R_{\text{ideal}}}, T_{\min}\right)
\]
– If the queue size is high, decrease the batch size and increase the interval to avoid overwhelming the queue:
\[
T_{\text{next}} = \min\left(\frac{B_{\text{next}}}{R_{\text{ideal}}}, T_{\max}\right)
\]
5. **Submit Videos to the Queue:**
– Submit `Bnext` videos to the queue every `Tnext` seconds.
6. **Feedback Loop:**
– Continuously monitor the queue size and VM processing speed to adjust the batch size and submission interval dynamically.
### Summary of Key Points:
– **Adaptive Batch Size:** The batch size of videos submitted to the queue is dynamically adjusted based on the queue's load and the VMs' processing speed.
– **Rate-Limiting:** Submission intervals are adjusted to avoid overwhelming the queue, ensuring a steady flow of videos that matches the VMs' processing capacity.
– **Real-Time Monitoring:** The algorithm continuously monitors the queue and adjusts parameters to maintain optimal performance.
This dynamic approach ensures that the video downloads are maximized without overwhelming the queue, leading to efficient utilization of the VMs.
The second run did as well:
To manage the download of millions of MP4 videos efficiently without overwhelming the queue, you can implement an adaptive load-balancing algorithm that dynamically adjusts the rate at which videos are submitted to the queue based on the current load and processing speed of the VMs.
### Algorithm: Adaptive Video Submission
#### 1. **Initial Setup:**
– **Initial Batch Size (`B0`)**: Start with an initial batch size of videos to submit to the queue.
– **Max and Min Batch Size (`Bmax`, `Bmin`)**: Define the upper and lower limits for the batch size.
– **Initial Submission Interval (`T0`)**: Start with an initial time interval between submissions.
– **Max and Min Interval (`Tmax`, `Tmin`)**: Define the upper and lower limits for the submission interval.
– **Threshold Queue Size (`Qthreshold`)**: Set a threshold for the queue size that indicates the queue is becoming too full.
#### 2. **Monitor the System:**
– **Queue Size (`Q`)**: Continuously monitor the current size of the queue (i.e., the number of videos waiting to be downloaded).
– **VM Throughput (`S`)**: Measure the average download rate of the VMs in terms of videos downloaded per second.
– **VMs Running Threads (`N`)**: Keep track of the number of active VMs and their respective threads.
#### 3. **Calculate Ideal Submission Rate:**
– Calculate the **ideal submission rate** to match the processing capacity of the VMs:
\[
R_{\text{ideal}} = S \times N \times \text{Threads per VM}
\]
– This rate represents the optimal number of videos that should be in the queue to keep all VMs busy without causing overload.
#### 4. **Adjust Batch Size and Submission Interval:**
– **Dynamic Batch Size Adjustment**:
– If the queue size `Q` is below a certain level (e.g., below `Qthreshold / 2`), **increase** the batch size to keep the VMs fully utilized:
\[
B_{\text{next}} = \min\left(B_{\text{current}} \times 1.5, B_{\text{max}}\right)
\]
– If the queue size `Q` exceeds the threshold, **reduce** the batch size to prevent overloading:
\[
B_{\text{next}} = \max\left(B_{\text{current}} \times 0.5, B_{\text{min}}\right)
\]
– **Dynamic Submission Interval Adjustment**:
– If the queue is getting too full (`Q > Qthreshold`), **increase** the time between submissions:
\[
T_{\text{next}} = \min\left(T_{\text{current}} \times 1.5, T_{\text{max}}\right)
\]
– If the queue is underutilized (`Q < Qthreshold / 2`), **decrease** the submission interval to speed up processing:
\[
T_{\text{next}} = \max\left(T_{\text{current}} \times 0.5, T_{\text{min}}\right)
\]
#### 5. **Submission Process:**
– **Submit `Bnext` videos** to the queue every `Tnext` seconds based on the dynamic adjustments.
– **Continuous Feedback Loop**: Continuously monitor the queue size and VM throughput, adjusting the batch size and submission interval in real-time to maintain optimal performance.
#### 6. **Handling New Videos:**
– **Prioritize or Integrate New Videos**: Depending on the importance of the new videos, either integrate them into the current queue or prioritize them in a separate, smaller batch that gets inserted at regular intervals.
### Summary:
– **Dynamic Rate Adjustment:** The batch size and submission interval are continuously adjusted based on real-time monitoring of the queue and VM throughput.
– **Balanced Queue Load:** The algorithm ensures that the queue is neither too full nor too empty, maintaining a steady flow of downloads.
– **Efficiency and Scalability:** By dynamically adapting to changing conditions, the algorithm maximizes the efficiency of the VMs without overwhelming the system.
This adaptive approach should help you efficiently manage the download process, ensuring that all videos are downloaded as quickly as possible without overloading the central queue.
What if we give ChatGPT the correct answer and ask its thoughts?
Would it make sense to use a trivially simple algorithm like a loop that checks the queue size, adds X new records, sleeps a few seconds and repeats the process to maintain a fixed queue size?
It accepts our answer, but pushes back on it somewhat:
Yes, using a trivially simple algorithm like a loop that checks the queue size, adds a fixed number of records (`X`), sleeps for a few seconds, and repeats the process can be effective in maintaining a fixed queue size. This approach, often referred to as a "constant fill" or "drip-feeding" algorithm, can work well if the system is relatively stable and the processing speed of the VMs is consistent.
### How It Would Work:
1. **Initialize Parameters:**
– **Desired Queue Size (`Qdesired`)**: The target number of videos you want to maintain in the queue.
– **Batch Size (`X`)**: The number of new records to add to the queue in each iteration.
– **Sleep Interval (`T`)**: The time in seconds to wait between each iteration.
2. **Algorithm Loop:**
– **Step 1:** Check the current size of the queue (`Qcurrent`).
– **Step 2:** If `Qcurrent` is less than `Qdesired`, add `X` new records to the queue.
– **Step 3:** Sleep for `T` seconds.
– **Step 4:** Repeat the process.
### Example Pseudocode:
"`python
Qdesired = 1000 # Target queue size
X = 100 # Number of videos to add in each loop
T = 5 # Sleep time in seconds
while True:
Qcurrent = check_queue_size() # Function to get current queue size
if Qcurrent < Qdesired:
add_to_queue(X) # Function to add X videos to the queue
time.sleep(T)
"`
### Considerations:
1. **Simplicity:** This approach is simple and easy to implement. It requires minimal real-time monitoring and adjustment.
2. **Stability:** If the VMs have a fairly constant processing rate, this method can maintain a steady queue size without overwhelming the system.
3. **Queue Size Adjustment:** You might need to tweak the parameters (`Qdesired`, `X`, `T`) to find the right balance for your specific workload and infrastructure.
4. **Handling Variability:** If there is significant variability in download speeds or processing times, this method may either underfill or overfill the queue. In such cases, more dynamic adjustments (like those described in more complex algorithms) might be necessary.
5. **Scalability:** This approach can work well at scale as long as the parameters are tuned correctly and the system’s performance remains stable.
### When to Use This Approach:
– **Predictable Workload:** If the system has a predictable and stable workload where the download speed and processing capacity of the VMs don't fluctuate significantly.
– **Simpler Implementation:** When you prefer a simple and straightforward implementation without the need for dynamic adjustments.
– **Quick Setup:** If you need to implement a solution quickly and can afford to manually adjust parameters if necessary.
### When a More Dynamic Approach Might Be Needed:
– **Highly Variable Workload:** If the system's workload is highly variable, with frequent changes in download speed or VM availability.
– **Maximizing Efficiency:** If you need to maximize the utilization of the VMs and ensure the fastest possible download times, a more sophisticated algorithm that dynamically adjusts to real-time conditions might be more appropriate.
In summary, a simple loop with a fixed queue size can be an effective and easy-to-implement solution in many cases, especially if the system is relatively stable. However, it’s essential to monitor the system's performance and be ready to adjust the parameters or adopt a more dynamic approach if needed.